Table Of Content
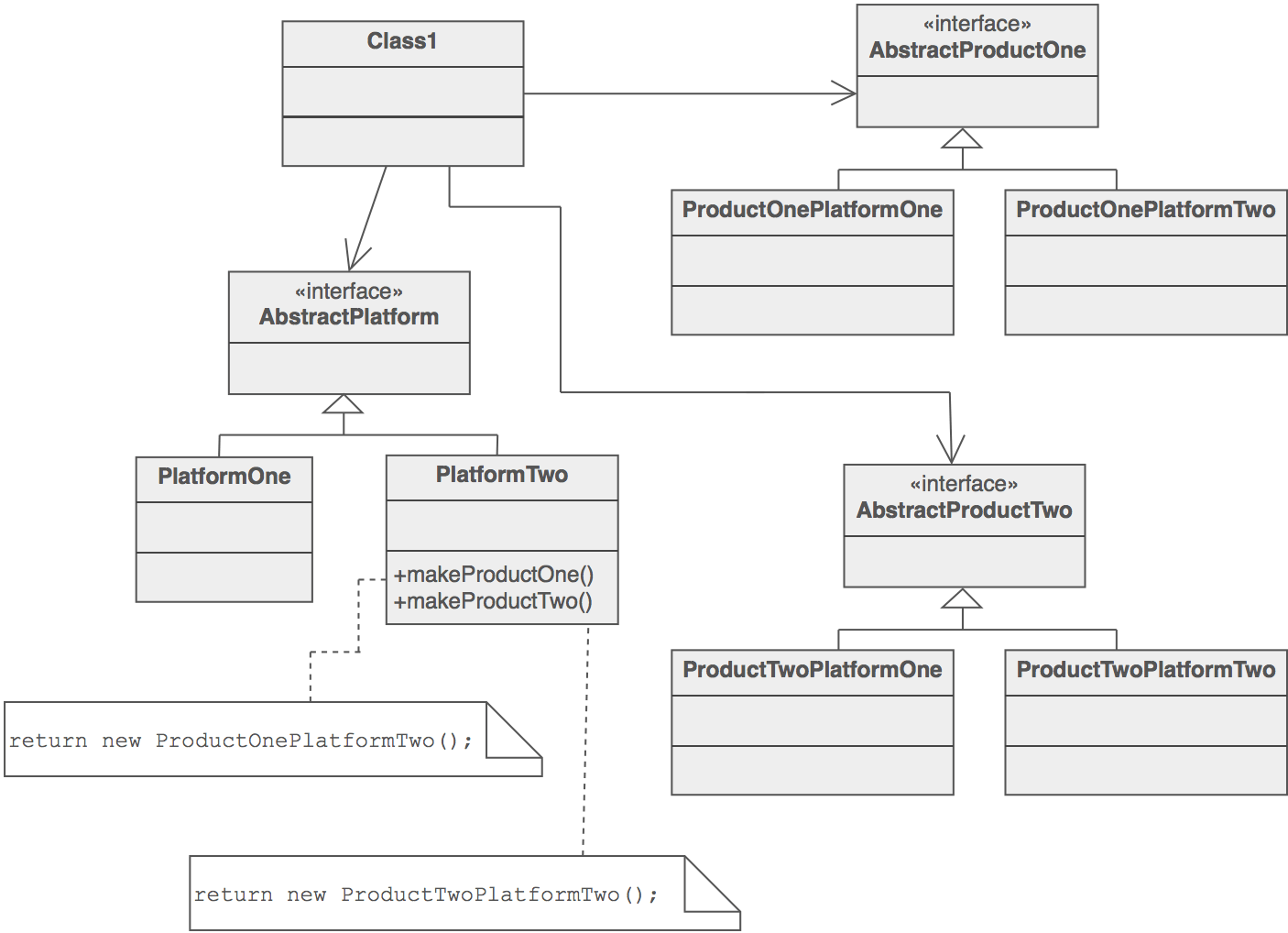
A factory is a class that returns products of a particular kind. For example, the ClassicFurnitureFactory can only create an Armchair, a CoffeeTable and Sofa objects. The client code calls the creation methods of a factory object instead of creating products directly with a constructor call (new operator). Since a factory corresponds to a single product variant, all its products will be compatible. In the above UML class diagram, the Client class that requires ProductA and ProductB objects does not instantiate the ProductA1 and ProductB1 classes directly. Instead, the Client refers to the AbstractFactory interface for creating objects, which makes the Client independent of how the objects are created (which concrete classes are instantiated).

Related patterns
The same UI elements in a cross-platform application are expected to behave similarly, but look a little bit different under different operating systems. Moreover, it’s your job to make sure that the UI elements match the style of the current operating system. You wouldn’t want your program to render macOS controls when it’s executed in Windows. When you buy from TSI you don't have to worry about the import process. Our knowledge of U.S. laws, customs, and logistics can be a valuable tool when you're importing your items to the U.S. While at MIT, he moved towards abstract painting and developed a parallel interest in new scientific imagery.
Client
Adding a new class to the program isn’t that simple if the rest of the code is already coupled to existing classes. Now we have the abstract factory that lets us make a family of related objects i.e. elven kingdom factory creates elven castle, king and army, etc. First of all, we have some interfaces and implementation for the objects in the kingdom. After you’ve created your account, you will start the quiz right away. Make sure to dedicate the necessary time to assessing your technical skills.
I thought factory methods only create one product?
Even though the coding examples will be in C++ as I am more comfortable with, they can be easily adjusted to any programming language like Java, Python, C#, Javascript and more. The Abstract Factory pattern first suggests explicitly declaring interfaces for each product in the product family (e.g., Armchair, Sofa, or CoffeeTable). Then we can make all variants of products follow those interfaces. For example, all Armchair variants can implement the Armchair interface; all Sofa variants can implement the Sofa interface, and so on. Having a factory for every single concrete implementation of computer is not a good idea and it will not be a good example to project abstract factory. The first thing the Abstract Factory pattern suggests is to explicitly declare interfaces for each distinct product of the product family (e.g., chair, sofa or coffee table).
Why is there a Creator and a Client?
Factory Method is a creational design pattern that provides an interface for creating objects in a superclass, but allows subclasses to alter the type of objects that will be created. The Factory Method pattern allows subclasses to choose the type of objects to create without revealing the creation process to the client. It provides an interface for creating objects in a way that is convenient and flexible for software development. When discussing Abstract Factory everything should be relevant to the keywords above.
Object-oriented vs. functional programming – O'Reilly - O'Reilly Media
Object-oriented vs. functional programming – O'Reilly.
Posted: Tue, 16 Feb 2016 08:00:00 GMT [source]
The abstract factory pattern is one of the classic Gang of Four creational design patterns used to create families of objects, where the objects of a family are designed to work together. In the abstract factory pattern you provide an interface to create families of related or dependent objects, but you do not specify the concrete classes of the objects to create. From the client point of view, it means that a client can create a family of related objects without knowing about the object definitions and their concrete class names.
The challenge is to find a suitable solution to create these types of Banking Accounts. The Factory Design Pattern is a promising candidate because its purpose is to manage the creation of objects as required. A default implementation can be provided, subclasses can still override the method to provide their own implementation.
The abstract factory defines an interface for creating buttons and checkboxes. There are two concrete factories, which return both products in a single variant. The client code has to work with both factories and products via their respective abstract interfaces. This lets you change the type of a factory that you pass to the client code, as well as the product variant that the client code receives, without breaking the actual client code. The Factory Design Pattern is a powerful technique for flexible object creation. By encapsulating the creation logic in a factory class, we achieve loose coupling and enhance the maintainability of our code.
You give the measurement for the door and your requirements, and he will construct a door for you. Your specifications are inputs for the factory, and the door is the output or product from the factory. For more information about Abstract factory method, refer this article. First of all, it doesn't happen that often that you have a set of interrelated types you want to instantiate. Abstract factory or any factory for that matter, they exist to solve the same problem, i.e, "Abstraction of object creation".
This type of design pattern comes under creational pattern as this pattern provides one of the best ways to create an object. As you can see, the creation of objects in the method create(AccountType type) depends on the constructor of the sub-classes. Therefore, if the constructor has many parameters, the factory method can become complex. Another rather intriguing motivation in this line of thoughts is a case where every-or-none of the objects from the whole group will have a corresponding variant. Based on some conditions, either of the variants will be used and in each case all objects must be of same variant.
Abstract Factory is a creational design pattern that provides an interface for creating families of related or dependent objects without specifying their concrete classes. Client code works with factories and products only through their abstract interfaces. This lets the client code work with any product variants, created by the factory object.